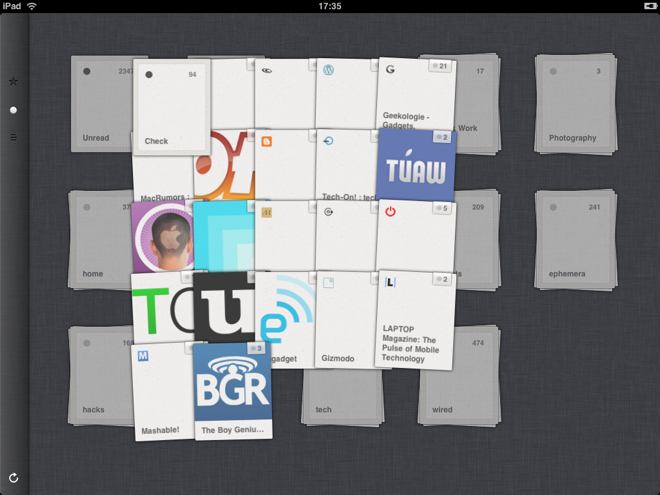


Over the past week there has been quite a bit of news coming out of the Twitter HQ. First it was announced that Twitter has acquired Tweetie and subsequently hired it's author Loren Brichter. They also have launched the promoted tweet service and for you real bird watchers out there the ability to add meta data to tweets using the API. Finally, it was confirmed that Twitter is going to launch their own URL shortener and stop using bit.ly. A recently published article on Mashable summaries one tech startup's frustration with the announcement of Twitter now coming out with it's own "official" Twitter client. Why is there so much frustration? Because developers, at times, feel they have been sabotaged by the very companies they are using. Just to be clear, they are upset because a service that Twitter owns, that they allow developers to use for free, is now being used by the very company that created it in the first place to promote their own brand!
I'm just saying...
I am developer and know what it is like to have that great idea or great app and to have some MUCH bigger/better company come along and launch their own version which inevitably kills mine before it got off the ground. However, those are the ropes. Don't put all your "1"'s and "0"'s into one app or service. That goes for everything in life. This animosity towards Twitter, and any other information service, is quite misdirected. As mentioned earlier, Twitter didn't go out and build their own app, they chose, quite possible the best Twitter client in the AppStore today. Did developers really expect Twitter to NOT have an official app. I am surprised it has taken them this long. That is like Apple releasing the iPod, but not iTunes.
What does that really mean? If you create something that is TRULY great then there is a good possibility that it will get picked up. Just look at all the startups and other mashups that have been acquired by big companies lately. Twitter has just released a site for developers that will aid the creation of new mashups and services to use with the information they provide not hinder innovation.
It is an unfortunate price that one has to pay when the service you rely on for your mashup comes out with their own official app that does the same thing and my sympathies are definitely with you, but if all you do is rely on that ONE great idea then you weren't going to last that long anyway.
Adding followers to a user one at a time is sometimes a VERY lengthy process. Last night the question was posed to me if there was a way to automate adding followers from users who follow someone else.
Example scenario:John Doe follows Jane Doe
John Doe wants to follow at 600 followers of Jane DoeUnfortunately, the Zend_Service_Twitter class doesn't offer any functionality to retrieve a list of followers from another user, but I was able to extend the class and add the functionality. The particular user that I chose to test has roughly 6,000 users. My client didn't want all 6,000, but the first 200. Within 10 minutes the new custom class was written, the script ran, and now my client was now following 200 new people of like mind. :) I plan on submitting the feature request to the Zend Framework gurus to have the ability to find followers from other users so that it can be apart of the main service.Until then here is the class and script: